[SPA Tutorial : Vue.js] Part1 : Project setting, gọi data API sử dụng Axios - Price

Step 1: Project Setting
[Ready] Node.js, Vue CLI phải được cài đặt đúng với OS của môi trường tiến hành.Tạo một dự án dựa trên phiên bản mới nhất của Vue CLI. (cài đặt Vue CLI)
Tham khảo các cài đặt bên dưới và tiến hành cài đặt Preset bằng Manual Mode.
vue create live-cryptocurrency
Vue CLI v3.3.0
┌───────────────────────────┐
│ Update available: 3.7.0 │
└───────────────────────────┘
? Please pick a preset: Manually select features
? Check the features needed for your project: Babel, Router, CSS Pre-processors, Linter
? Use history mode for router? (Requires proper server setup for index fallback in production) No
? Pick a CSS pre-processor (PostCSS, Autoprefixer and CSS Modules are supported by default): Sass/SCSS
? Pick a linter / formatter config: Basic
? Pick additional lint features: (Press <space> to select, <a> to toggle all, <i> to invert selection)Lint on save
? Where do you prefer placing config for Babel, PostCSS, ESLint, etc.? In dedicated config files
? Save this as a preset for future projects? No
✨ Creating project in D:\front-end\vue\live-cryptocurrency.
🗃 Initializing git repository...
⚙ Installing CLI plugins. This might take a while...
📄 Generating README.md...
🎉 Successfully created project live-cryptocurrency.
👉 Get started with the following commands:
$ cd live-cryptocurrency
$ npm run serve
Nếu lệnh npm run serve được tiến hành bình thường thì phía console sẽ hiện thông báo như sau.DONE Compiled successfully in 12898ms 17:37:17
App running at:
— Local: http://localhost:8080/
— Network: http://192.168.0.xxx:8080/
Note that the development build is not optimized.
To create a production build, run npm run build.
Nếu bạn truy cập vào đường dẫn http://localhost:8080/ sẽ nhìn thấy màn hình Welcome như bên dưới được thiết lập mặc định cho project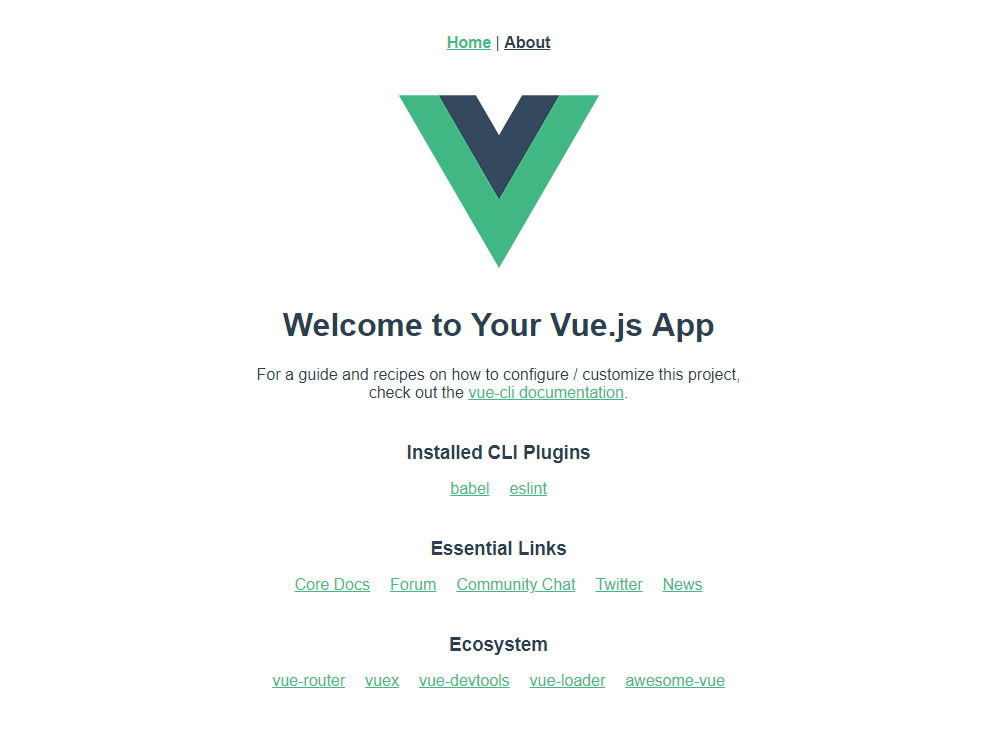
Step 2: Yêu cầu API
Tôi dự định yêu cầu và hiển thị dữ liệu về giá cả theo đồng won (KRW) và tỷ lệ biến động của của Bitcoin (BTC), Ethereum (ETH) và Ripple (XRP) dưới dạng bảng trên màn hình.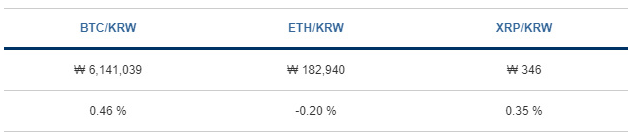
Open API : Cryptocompare.com (Price, Ratio, News)
Request URL: https://min-api.cryptocompare.com/data/pricemultifull?fsyms=BTC,ETH,XRP&tsyms=KRW
Nhập Request URL vào vùng nhập phía trên của màn hình bên dưới và chọn nút Execute Call.
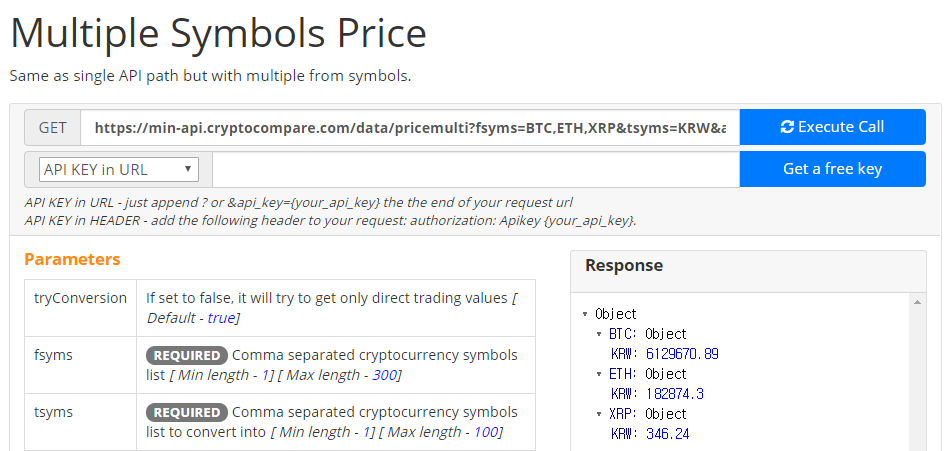
Sau khi hoàn thành quá trình đăng ký và login, hãy chọn lại nút Get a free key để nhận API Key và thực hiện yêu cầu bên dưới.
https://min-api.cryptocompare.com/data/pricemultifull?fsyms=BTC,ETH,XRP&tsyms=KRW&api_key=발급받은API Key
Vì đó là yêu cầu GET nên bạn có thể kiểm tra ngay trong trình duyệt.
[Response Data Sample]
{
"RAW": {
"BTC": {
"KRW": {
"PRICE": 6130238.03,
"CHANGEPCT24HOUR": 0.06215658987244043
}
},
"ETH": {
"KRW": {
"PRICE": 182889.95,
"CHANGEPCT24HOUR": -0.4627782718931592
}
},
"XRP": {
"KRW": {
"PRICE": 346.22,
"CHANGEPCT24HOUR": -0.1586065691957074
}
}
},
"DISPLAY": {
"BTC": {
"KRW": {
"PRICE": "₩ 6,130,238.0",
"CHANGEPCT24HOUR": "0.06"
}
},
"ETH": {
"KRW": {
"PRICE": "₩ 182,890.0",
"CHANGEPCT24HOUR": "-0.46"
}
},
"XRP": {
"KRW": {
"PRICE": "₩ 346.22",
"CHANGEPCT24HOUR": "-0.16"
}
}
}
}
Cấu trúc data Response có định dạng JSON và được hình thành bởi cặp data ở hai định dạng Raw và Display.Thông thường, Raw data được xử lý ở Front-end và được format theo định dạng do màn hình cung cấp, tuy nhiên nhận thấy rằng data được cung cấp bằng cả hai định dạng thì có thể giảm được khó khăn trong việc xử lý hậu kỳ.
Bây giờ bạn đã hoàn thành việc phát hành API Key và data call test, đã đến lúc tiến hành áp dụng tiêu chuẩn code.
Step 3: Xây dựng code
Để xử lý các yêu cầu API, tôi cài đặt Axios library được sử dụng rộng rãi mà không hề phân biệt Vue.js, React.npm install axios --save
/App.vue
<template>
<div id="app">
<router-view/>
</div>
</template>
<style lang="scss">
#app {
font-family: 'Avenir', Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #2c3e50;
}
#nav {
padding: 30px;
a {
font-weight: bold;
color: #2c3e50;
&.router-link-exact-active {
color: #42b983;
}
}
}
</style>
Vì đã cài đặt router trong project setting nên tôi khớp path và component được xác định trong router.js rồi hiển thị nội dung trong khu vực <router-view/>./router.js
import Vue from 'vue'
import Router from 'vue-router'
import Home from './views/Home.vue'
Vue.use(Router)
export default new Router({
routes: [{
path: '/',
name: 'home',
component: Home,
meta: {
title: 'Live Cryptocurrency'
}
}]
})
/src/views/Home.vue
<template>
<div class="home">
<Cryptocurrency/>
</div>
</template>
<script>
import Cryptocurrency from '@/components/Cryptocurrency.vue'
export default {
name: 'home',
components: {
Cryptocurrency
}
}
</script>
Tôi đang gọi component Cryptocurrency.vue trong Home.vue có trong folder Views./src/components/Cryptocurrency.vue
<template>
<div>
<header>
<h1>Live Cryptocurrency</h1>
</header>
<article>
<h2>Live Prices</h2>
<table>
<caption>Live Cryptocurrency</caption>
<thead>
<tr>
<th>BTC/KRW</th>
<th>ETH/KRW</th>
<th>XRP/KRW</th>
</tr>
</thead>
<tbody>
<tr>
<td>{{cryptoPrice.BTC}}</td>
<td>{{cryptoPrice.ETH}}</td>
<td>{{cryptoPrice.XRP}}</td>
</tr>
</tbody>
</table>
</article>
</div>
</template>
<script>
// Add modules
import axios from 'axios'
// Singl file component scope
export default {
name: 'cryptocurrency',
// 데이터 객체
data() {
return {
cryptoPrice: {
BTC: 0,
ETH: 0,
XRP: 0
}
}
},
// Vue 인스턴스에 추가할 메소드
methods: {
// 암호 화폐 가격 정보 처리
bindData() {
axios
.get('https://min-api.cryptocompare.com/data/pricemultifull?fsyms=BTC,ETH,XRP&tsyms=KRW&api_key=발급받은API Key')
.then(res =>{
console.log('API Response : ', res);
const obj = Object.keys(this.cryptoPrice)
for(var i=0;i<obj.length;i++){
this.cryptoPrice[obj[i]] =
res.data.DISPLAY[obj[i]].KRW.PRICE
console.log(obj[i] + ' : ' + this.cryptoPrice[obj[i]]);
}
})
.catch(e => {
console.log(e);
})
}
},
// 컴포넌트, 템플릿, 렌더링된 돔에 접근할 수 있는 상태 (인스턴스 라이프사이클에 속함)
mounted() {
this.bindData()
},
}
</script>
<style lang="scss">
body {
min-width:360px;
margin:0
}
article {
padding:10px
}
.blind {
visibility: hidden;
position: absolute;
left: 0;
top: 0;
width: 1px;
height: 1px;
line-height: 0;
font-size: 0;
}
header {
height: 64px;
border-bottom: 2px solid #2a3b52;
background: #2e4564;
}
h1 {
margin: 0;
padding: 24px 0 0;
color: #fff;
font-size: 18px;
text-transform: uppercase;
}
h2 {
margin: 0;
padding: 6px 0 15px;
color: #333;
font-size: 16px;
font-weight: bold;
text-align: left;
text-transform: uppercase;
}
table {
width: 100%;
margin-bottom: 20px;
table-layout: fixed;
border-collapse: collapse;
border-top: 1px solid #ccc;
text-align: center;
font-size: 12px;
line-height: 1.5;
caption {
position: absolute;
left: 0;
top: 0;
width: 1px;
height: 1px;
font-size: 0;
line-height: 0;
}
}
thead th {
padding: 10px;
font-weight: 700;
vertical-align: top;
color: #369;
border-bottom: 3px solid #036;
}
tbody th {
width: 150px;
padding: 10px;
font-weight: 700;
vertical-align: top;
border-bottom: 1px solid #ccc;
background: #f3f6f7;
}
td {
width: 350px;
padding: 10px;
vertical-align: top;
border-bottom: 1px solid #ccc;
}
}
</style>
Cú pháp chính Cryptocurrency.vue
// ① HTML 템플릿 구문
<tr>
<td>{{cryptoPrice.BTC}}</td>
<td>{{cryptoPrice.ETH}}</td>
<td>{{cryptoPrice.XRP}}</td>
</tr>
// ② 데이터 객체
data() {
return {
cryptoPrice: {
BTC: 0,
ETH: 0,
XRP: 0
}
}
},
// ③ Vue 인스턴스에 추가할 메소드
methods: {
// 암호 화폐 가격 정보 처리
bindData() {
axios
.get(API Request URL)
.then(res => {
const obj = Object.keys(this.cryptoPrice)
for (var i = 0; i < obj.length; i++) {
this.cryptoPrice[obj[i]] =
res.data.DISPLAY[obj[i]].KRW.PRICE
}
.catch(e => {
console.log(e);
})
})
}
},
//컴포넌트, 템플릿, 렌더링된 돔에 접근할 수 있는 상태 (인스턴스 라이프사이클에 속함)
mounted() {
this.bindData()
},
Có thể binding ② đối tượng dữ liệu bằng cú pháp hai dấu ngoặc nhọn {{}} trong ① HTML template.Nếu thay đổi đối tượng dữ liệu thông qua ③ event và method, content được binding cũng được cập nhật.
[Dòng chảy Data]
- CryptoPrice.BTC, cryptoPrice.ETH, cryptoPrice.XRP trong đối tượng dữ liệu được khởi tạo lại bằng 0
- Giá trị được khởi tạo matching với vùng {{}} được gọi
- Tiến hành hàm bindprice () ở trạng thái mounted theo Vue lifecycle
- Trong hàm bindData (), gửi yêu cầu GET tới API bằng Axios và thực hiện cú pháp then nếu có giá trị kết quả, lỗi đầu ra trong cú pháp "catch" nếu xảy ra lỗi.
Trong cú pháp then, matching giá tri kết quả Ajax với tham số res rồi truy cập node DISPLAY > [BTC, ETH, XRP] > KRW > PRICE để update giá trị kết quả theo đinh dạng phù hợp với đầu ra trên đối tượng dữ liệu - Do update đối tượng dữ liệu nên content được matching trên HTML template cũng được update
Sản phẩm đã hoàn thiện các bạn có thể check tại trang http://fe.hivelab.co.kr/spa.
[▣] [Vue.js] SPA Tutorial - Part1
Project setting, gọi data API sử dụng Axios - Price
[Vue.js] SPA Tutorial - Part2
Gọi data API sử dụng Axios - Ratio, News
[Vue.js] SPA Tutorial - Part3
Control News data — More button
Comments
Post a Comment